Note: The requirements below must be met to get any credit for this assignment.
- Only the following imports are allowed.
- javax.swing.*
- java.awt.*
- java.util.ArrayList
- java.util.Scanner
- java.io.*
- In particular, you must implement all the code for sorting and searching yourself.
- Any sorting implemented must use the quicksort algorithm.
- Any searching implemented must use the binary search algorithm.
- Your code must be formatted neatly. Eclipse can do this for you.
- Your code must follow the conventions described here.
- Add Javadoc comments to your code and generate the Javadoc file before submitting your assignment. Javadoc comments must show who authored that piece of code.
All the items above must be done to get any credit on the assignment.
Assignment
Implement the popular word game Ruzzle, shown here.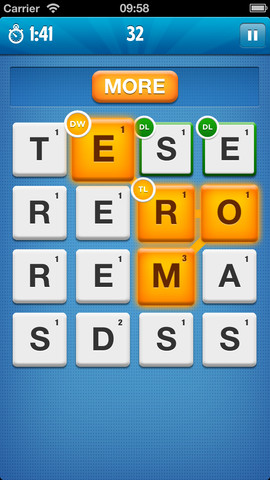
Minimum requirements for user interface
- Game board similar to the one pictured
- Letter tiles, showing letter and value of each tile. Here are the values of all the letters.
- Each tile has an optional indicator for double word score. Your indicator need not look like the one pictured.
- Each tile has an optional indicator for double letter score. Your indicator need not look like the one pictured.
- Menu with the options: Game (New, Read Word List, Exit)
- Two-minute timer - starts at 02:00 and counts the seconds down to 00:00
- Current score display - score is calculated using the values of letters used in a word, plus any applicable double letter and double word multipliers.
Functional Requirements
- When launched, the program does the following:
- Creates and displays a new game
- Starts the timer
- Waits for user to find words
- User selects letters to form words using the mouse. During this process:
- Selected letters are highlighted as they are selected.
- Word under construction is shown on the board
- When the word is completed:
- your program checks to see if proposed word is acceptable. A proposed word is acceptable only if it appears in this word list.
- This word list can be changed by adding words to the text file, or by
deleting words from it. Your program will read the word list when the
program is launched, or when the user selects Game/Read Word List. Your program must work correctly with any text file representing a list of words.
- Acceptance of a word is indicated by an "acceptance" sound. Rejection of a proposed word is indicated by a "rejection" sound.
- Game ends when all words have been found, or when the time runs out, whichever comes first. When
the game ends, your program displays a message like, "You found 24 out
of 152 words". It also shows the 24 words found and all the 152 words
that are contained in the puzzle.
Grading Rubric
- (15 points) Project demonstrates good object-oriented design.
- (15 points) The user interface described above.
- 15 points each for the 4 functional requirements listed above. Each element will be graded on a boolean basis. That is, you get full credit for that element if it fully meets the requirement.You get no credit for that element if any part of that requirement is not met.
- (10 points) Something you add to the project that is not required. How many points you get out of 10 depends on my perception of the utility and coolness of your addition.